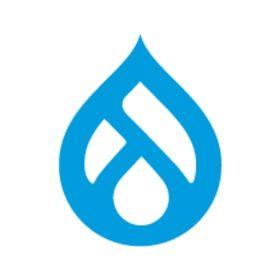
Hello,
I'm going to use the Date API of the Date Module to render a simple date widget.
There is not much documentation but the most helpful is: Date Form Elements
I created the following field definitions in the .install file and my code is based on the node_example:
function _event_installed_fields() {
$t = get_t();
return array(
'event_date' => array(
'field_name' => 'event_date',
'type' => 'date',
'settings' => array(
'tz_handling' => 'date',
),
),
);
}
function _event_installed_instances() {
$t = get_t();
return array(
'event_date' => array(
'field_name' => 'event_date',
'label' => $t('Der Zeitpunkt des Events.'),
'widget' => array(
'type' => 'date_select', // see https://drupal.org/node/1364748
),
'display' => array(
'event_list' => array(
'label' => 'hidden',
'type' => 'date_select',
),
),
),
);
}
This is my event_form() in .module:
function event_form($node, $form_state) {
$form = array();
$type = node_type_get_type($node);
// implementation of the title field
if ($type->has_title) {
$form['title'] = array(
'#type' => 'textfield',
'#title' => check_plain($type->title_label),
'#required' => TRUE,
'#default_value' => $node->title,
'#maxlength' => 255,
'#weight' => -5,
);
}
// Creating the date/time element
// Provide a default date in the format YYYY-MM-DD HH:MM:SS.
$date = date('Y-m-d');
// Provide a format using regular PHP format parts (see documentation on php.net).
// If you're using a date_select, the format will control the order of the date parts in the selector,
// rearrange them any way you like. Parts left out of the format will not be displayed to the user.
$format = 'd/m/Y';
$form['event_date'] = array(
'#type' => 'date_select', // types 'date_text' and 'date_timezone' are also supported. See .inc file.
'#title' => t('Zeitpunkt'),
'#default_value' => $date,
'#date_format' => $format,
'#date_timezone' => 'Europe/Berlin', // Optional, if your date has a timezone other than the site timezone.
'#date_label_position' => 'within', // See other available attributes and what they do in date_api_elements.inc
'#date_year_range' => '0:+3', // Optional, used to set the year range
);
return $form;
}
The field is created as text field in the database and the widget is correctly rendered. But if I save a new event I get the following errors:
Warning: array_values() expects parameter 1 to be array, string given in _field_filter_items() (line 527 of /var/www/drupal/modules/field/field.module).
Warning: Invalid argument supplied for foreach() in date_field_validate() (line 313 of /var/www/drupal/sites/all/modules/date/date.field.inc).
I think I'm doing something wrong and I think it's no problem in the module so far, but I don't see my mistake. I'm looking forward for help.
The documentation just says:
These are self-validating elements, you shouldn't need to do anything except include them in your form. You pass them parameters for the timezone, format, and a default value in the format YYYY-MM-DD HH:MM:SS, and it will convert your input into the format the element wants, split the date and time into different fields as needed, then accept and validate the user input and convert it back into a string in the same format you originally provided (YYYY-MM-DD HH:MM:SS). So you pass it a string and it will pass back a string by the time you get to your own validation function.
Thanks in advance!
Comments
Comment #1
KarenS CreditAttribution: KarenS commentedMajor problems are release blockers. A support question about custom code is not a major issue. I am focused on fixing bugs and finishing features that are release blockers. Questions about custom code won't get answered until after that is done.