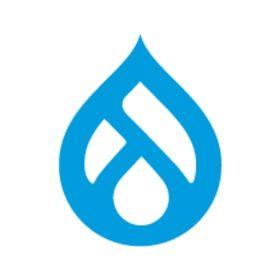
Problem/Motivation
Currently, the only way to interface with drupal from the command line is via a 3rd party module (drush). Because it's a 3rd party module, it can't be expected to keep up with the fast pace of changes in core.
By adding Command classes from Symfony to do tasks like site install, enable/disable modules and run tests, Core can test it's own command line tools and verify a new patch doesn't break them.
Here's a sample class skeleton for a command:
class SiteInstall extends Command
{
protected function configure()
{
// Configuration and Arguments go here.
}
protected function execute(InputInterface $input, OutputInterface $output)
{
// Commands to execute Site Install go here.
}
}
Proposed resolution
- Get Console Component in Core
- Write classes that extend Symfony\Component\Console\Command\Command to do tasks we decide Core should handle
- Write tests for each Command class we right that verify the Command works
- Possibly, create a simple script that users can run from CLI directly to do run these command classes written
Remaining tasks
User interface changes
API changes
Drush, or any other script can call these command classes in core directly using a snippet of code to execute the command:
$application = new Application();
$application->add(new SiteInstall());
$application->run();
Note: the class autoloader would need to be instantiated in there as well, just keeping the code as simple as possible at a conceptual level.
Comments
Comment #1
moshe weitzman CreditAttribution: moshe weitzman commentedThe Summary for this issue is pitiful. Please actually describe how you see Console fitting into Drush. Lets get technical.
Also, Drush "seems to be open source" so everyone is welcome to submit patches. We are a small team and we can't exactly keep up with the torrid pace that D8 is on right now.
I'm hopefully doing a session on 'Developing for Drush' at Syndney and I'll get into Drush architecture.
Comment #2
pounardI think the summary is off topic too, Drush is contrib. But I see plenty of good things with integrating the Console component into core! In many cases, this would have helped me in various projects.
Comment #3
disasm CreditAttribution: disasm commentedI'll update the issue summary next week, or close the issue out. For now I'm marking this postponed until I do some more research myself. I agree, drush is off topic for core, but having an API to interact from the CLI in core I think is worthwhile. chx mentioned in #drupal-contribute that there are already ways to do this without adding the console component.
Comment #4
disasm CreditAttribution: disasm commentedMy thoughts here were if Drupal Core defined it's own console commands, those could be tested in automated tests that verified they worked properly. That way if an API changed, it would cause errors right away and the author of the patch would know they broke compatibility with the command line interface. I'm not sold on using the Symfony Console Component, but I think it is crucial that core provides some standard interface for doing things like site install. I would like to here details from chx on how this could be done without the console component. To answer moshe on how the console component could help drush, the docs for the console component (http://symfony.com/doc/2.0/components/console/introduction.html) show creating a command for doing something (i.e. site install), and then creating a script that calls that command. My thoughts are the command class could be SiteInstall, and then drush or any other script could implement the script that runs the command. Then tests can be written for each command class core implements, and drush can "trust" that as long as it uses the command class to do a task, that Core takes responsibility for it working.
Comment #5
pounardHaving Symfony Console in Core, and implementing simple commands such as cache clear, CSS / JS rebuild, switch theme, show variable, dump config, etc... is probably a must have in core. I'm not speaking about advanced stuff here, just about simple developer helpers.
Comment #6
disasm CreditAttribution: disasm commentedComment #6.0
disasm CreditAttribution: disasm commentedUpdated issue summary.
Comment #7
bcw CreditAttribution: bcw commentedIt would be nice if developers had the choice of whether to register either new drush commands (using the drush api) or (SF2) ContainerAwareCommands for their cli tasks, and if there were a single point of entry for both APIs, even if perhaps there were a separate option for running CACs, e.g.:
$ drush --command cron:task
Symfony2 Commands come with a clean api for working with other Symfony2 components like the DIC, and for working with other Commands. It seems like a shame not to expose these interfaces to Drupal developers. In lieu of them, however, what would be the recommended path for providing a container-aware context for a cli task? A drush module?
Comment #8
Jaza CreditAttribution: Jaza commentedI think that it would make sense to re-engineer Drush for D8 on top of the Symfony2 Console component. Not sure if this has been proposed elsewhere already?
D8 core has been re-written to be built on top of Symfony2 components for many bits of functionality. So, it seems logical that many D8 contrib modules will also inevitably re-evolve upon a Symfony2 foundation.
However, putting Symfony2 Console in core, and/or moving parts of Drush into core, is a whole different topic (and a much more controversial one, I'd say).
As far as I'm aware, nobody has considered moving Drush (or any subset of Drush) into core... and I don't see why we'd want to do this, either.
Also, not sure I understand the argument about testing being put forward here. Why does the Console component need to be in core, in order to be testable? Drupal core already has excellent unit test coverage, I don't see how adding command-line functionality tests to core will improve this. Can't we just add Console as the foundation of Drush, and then write more tests for Drush?
Comment #9
disasm CreditAttribution: disasm commentedThe idea is that core would have it's own console commands (independent of drush) for doing coreish tasks, like site install, enabling/disabling modules, etc... If these console commands are in core, then theoretically simpletests could be written that would cause a failure if an API change broke a console command. Drush could optionally, if it chose to, instead of implementing it's own bootstrap, use the core console commands, and expand on them for contrib module type tasks.
That being said, talking with people in #drupal-contribute, if this happens, it's not going to until D9, so marking postponed to end the discussion until then.
Comment #10
Letharion CreditAttribution: Letharion commentedI'm making a bold move here, moving over to Drush, and suggest that #8 is an interesting idea.
I think could make sense to a least _consider_ using Symfony for Drush functionality, based on the same principles as Core.
Whenever we share problem-space with a larger community than "only" Drupal, we should attempt to solve it in a shared way. I have aboslutely zero information about the command component, but I does sound like it's worth looking into more.
As is often otherwise the case for Core, if this works in Contrib-space, it's easier to consider moving it into Core.
If the Drush maintainers find this an utterly horrible idea, just revert the title and project change. :)
Comment #11
greg.1.anderson CreditAttribution: greg.1.anderson commentedI agree that the Drush queue is the best place to discuss using the Symfony Console component in Drush, and that discussion is a good precursor to a future discussion (in a separate thread) on Drush in core, or a subset of Drush in core.
The Drush maintainers have discussed different ways that object-oriented components could be introduced into Drush, and there are, in fact, already a few places that Drush uses classes. Regarding the use of Symfony components, see #1316322: Add PSR-0 autoloader to drush and #1487654: Drush object-oriented context and commandfile design.
I think that #7 is a good idea; however, as was mentioned in #2, we need specific suggestions on how the Console component could improve Drush, or make it easier for Drush to interoperate with Drupal 8. To move things forward, we need people making very specific technical recommendations, or better yet, submitting patches.
Comment #12
greg.1.anderson CreditAttribution: greg.1.anderson commentedThis issue was marked
closed (won't fix)
because Drush has moved to Github.If this feature is still desired, you may copy it to our Github project. For best results, create a Pull Request that has been updated for the master branch. Post a link here to the PR, and please also change the status of this issue to
closed (duplicate)
.Please ask support questions on Drupal Answers.
Comment #13
greg.1.anderson CreditAttribution: greg.1.anderson commentedThe GitHub issue is https://github.com/drush-ops/drush/pull/88
Comment #13.0
greg.1.anderson CreditAttribution: greg.1.anderson commentedUpdated issue summary.