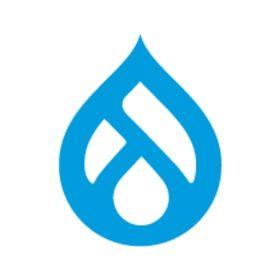
By ayush.sk on
Hello,
i was working on solution or task where we have a paragraph type and in that we are using a dynamic entity reference field ,
while adding a entries we are able to add duplicate entries.So, is there any solution or module that can help to restrict the user to add duplicate entries in the same field.
Comments
To prevent duplicate entries
To prevent duplicate entries in a dynamic entity reference field in Drupal, you can use form validation to check for duplicates before the form is submitted. Here’s a custom solution using hook_form_alter() and a custom validation function:
use Drupal\Core\Form\FormStateInterface;
use Drupal\Component\Utility\NestedArray;
/**
* Implements hook_form_alter().
*/
function mymodule_form_alter(&$form, FormStateInterface $form_state, $form_id) {
// Check if the target field is in the form.
if (isset($form['your_entity_reference_field'])) {
// Add a custom validation handler.
$form['#validate'][] = 'mymodule_validate_no_duplicates';
}
}
/**
* Custom validation handler to prevent duplicates.
*/
function mymodule_validate_no_duplicates(array &$form, FormStateInterface $form_state) {
// Get the user input from the form state.
$input = $form_state->getValue('your_entity_reference_field');
$ids = array_column($input, 'target_id');
// Remove any empty values and count the unique IDs.
$ids = array_filter($ids);
if (count($ids) !== count(array_unique($ids))) {
// Set an error if there are duplicate values.
$form_state->setErrorByName('your_entity_reference_field', t('Duplicate entries are not allowed.'));
}
}
Replace 'your_entity_reference_field' with the machine name of your entity reference field. This code snippet will add a custom validation function that checks for duplicate target IDs in the entity reference field and sets a form error if duplicates are found. DogNearMe
If you prefer a module solution, you might want to look into the Unique Field module or similar contributed modules that provide this functionality. However, writing a custom validation function as shown above gives you more control and can be tailored to your specific needs. Remember to replace 'mymodule' and 'your_entity_reference_field' with the appropriate names from your project.