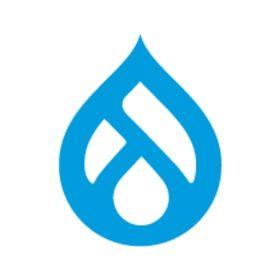
By Begun on
Hi All,
I am trying to create a function which deletes user created nodes when I delete a given user. I have a function which I call in hook_user(), but it doesn't seem to work. Every time I delete a user, the nodes they created remain; furthermore, the uid of these nodes are all set to 0. Assuming there is nothing wrong with my function, I am guessing that the uids of these nodes is being changed to 0, before my function has time to run.
Is there a way to preprocess nodes of a given uid before the user is deleted?
Regards,
Begun
function namecards_user($op, &$edit, &$account, $category = NULL) {
switch ($op) {
case 'insert':
// Only create settings for user with permission to use namecards module.
if (user_access('access namecards', $account)) {
_namecards_user_settings_add($account->uid);
drupal_set_message('Namecard user ' . $account->uid . ' settings added');
}
break;
case 'delete':
// TODO: Check if user has contacts in system and process these contacts before deleting user.
drupal_set_message('UID: ' . $account->uid);
$delete_users_contacts = variable_get('namecards_delete_user_contacts_options', 0);
_namecards_delete_users_contacts($account->uid, $delete_users_contacts);
// Remove namecard settings for a deleted user.
_namecards_user_settings_delete($account->uid);
break;
case 'login':
_namecards_user_settings_set_session_var(TRUE);
break;
case 'logout':
if (isset($_SESSION['namecards_user_namecard_settings'])) {
_namecards_user_settings_set_session_var(FALSE);
}
break;
}
}
function _namecards_delete_users_contacts($uid, $option = 0) {
switch ($option) {
case 0:
$sql = '
SELECT
n.nid
FROM
{node} n
WHERE
n.type = "namecard_namecard"
AND
n.uid = %d
';
break;
case 1:
$sql = '
SELECT
n.nid
FROM
{node} n
LEFT JOIN
{content_type_namecard_namecard} ctnn ON n.nid = ctnn.nid
WHERE
n.type = "namecard_namecard"
AND
n.uid = %d
AND
ctnn.field_public_value = 0
';
break;
}
$result_set = db_query($sql, $uid);
while ($result = db_fetch_object($result_set)) {
node_delete($result->nid);
}
}
Comments
Any ideas anyone?
Any ideas anyone?
At a first glance, your
At a first glance, your function looks fine, and I think your hypothesis about the node ids being set to zero is probably correct. I suggest trying one of the following two things.
1) Delete the nodes in hook_user() when $op equals validate. You will have to check that the clicked button was the delete button. This will probably work, however it's a little hacky, as you should really only be validating things in the validate function, and could cause problems if the deletion of the user fails after this.
2) Attach a custom submit function to the delete button in hook_form_alter(). This function should be run before the regular form submit function is run (I believe). This method is a better more proper way to do it, but will take a little more coding.
Thank you Jay
I decided to go with the second suggestion. For the benefit of others this is what I did:
Add an additional submit function to form 'user_multiple_delete_confirm' using hook_form_alter(). This is the ID of the form responsible for deleting one or more users.
More complete workaround
I've made a comment about this behavior in the API page for
hook_user()
. That comment also includes a workaround usinghook_form_alter()
which was built using your example, but also includes the user_confirm_delete form when a user is deleted from the Edit Account page.