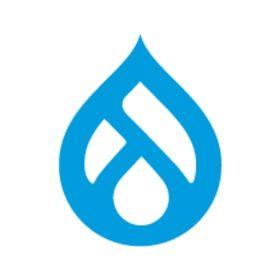
The description/help text on fields in a node form are always inconveniently placed below the actual field so if you have a particularly long field (or several fields when it's a multi-value field) a user may not see the description/help text right away.
The following code will move the description to just below the field label in both single and multi-value fields. It should be placed in template.php
<?php
function YOUR_THEME_NAME_form_element($element, $value) {
$output = '<div class="form-item"';
if (!empty($element['#id'])) {
$output .= ' id="'. $element['#id'] .'-wrapper"';
}
$output .= ">\n";
$required = !empty($element['#required']) ? '<span class="form-required" title="'. t('This field is required.') .'">*</span>' : '';
if (!empty($element['#title'])) {
$title = $element['#title'];
if (!empty($element['#id'])) {
$output .= ' <label for="'. $element['#id'] .'">'. t('!title: !required', array('!title' => filter_xss_admin($title), '!required' => $required)) ."</label>\n";
}
else {
$output .= ' <label>'. t('!title: !required', array('!title' => filter_xss_admin($title), '!required' => $required)) ."</label>\n";
}
}
if (!empty($element['#description'])) {
$output .= ' <div class="description">'. $element['#description'] ."</div>\n";
}
$output .= " $value\n";
$output .= "</div>\n";
return $output;
}
?>
The above code takes care of single value fields. Following code is for multi-value forms:
<?php
function YOUR_THEME_NAME_content_multiple_values($element) {
$field_name = $element['#field_name'];
$field = content_fields($field_name);
$output = '';
if ($field['multiple'] >= 1) {
$table_id = $element['#field_name'] .'_values';
$order_class = $element['#field_name'] .'-delta-order';
$required = !empty($element['#required']) ? '<span class="form-required" title="'. t('This field is required.') .'">*</span>' : '';
$header = array(
array(
'data' => t('!title: !required', array('!title' => $element['#title'], '!required' => $required)).'<br /><div class="description">'. $element['#description'] .'</div>',
'colspan' => 2
),
t('Order'),
);
$rows = array();
// Sort items according to '_weight' (needed when the form comes back after
// preview or failed validation)
$items = array();
foreach (element_children($element) as $key) {
if ($key !== $element['#field_name'] .'_add_more') {
$items[] = &$element[$key];
}
}
usort($items, '_content_sort_items_value_helper');
// Add the items as table rows.
foreach ($items as $key => $item) {
$item['_weight']['#attributes']['class'] = $order_class;
$delta_element = drupal_render($item['_weight']);
$cells = array(
array('data' => '', 'class' => 'content-multiple-drag'),
drupal_render($item),
array('data' => $delta_element, 'class' => 'delta-order'),
);
$rows[] = array(
'data' => $cells,
'class' => 'draggable',
);
}
$output .= theme('table', $header, $rows, array('id' => $table_id, 'class' => 'content-multiple-table'));
$output .= drupal_render($element[$element['#field_name'] .'_add_more']);
drupal_add_tabledrag($table_id, 'order', 'sibling', $order_class);
}
else {
foreach (element_children($element) as $key) {
$output .= drupal_render($element[$key]);
}
}
return $output;
}
?>
You will also have to do a little bit of css since the description in multi-value fields will be bold.
The following css should do it (goes in theme css file of course):
form#node-form .description {
font-weight: normal!important;
font-style: italic!important;
font-size: 12px!important;
}
You can adjust the css as needed.
Comments
It worked but the description for password is above the title
I just put the code only with the change of theme name and worked for me. Thanks!
Only the thing is that the description for password in "account information" on user/%/edit is above the title but not under the title.
How can I fix this?
Works sometimes
This broke the datepicker for date fields using the popup calendar for selecting date. Not sure what other js field validations or functions could be affected. This needs more peer review.
A better approach can be found here: http://groups.drupal.org/node/206593#comment-747503
I've written a module for
I've written a module for Drupal 7 that offers a solution to this problem:
http://drupal.org/project/label_help
----------------
Customer Support Engineer, Granicus
https://granicus.com
Solution is not the same as the problem
It's probably worth pointing out that Sheldon's Label Help module doesn't do exactly what is attempting to be solved here, as it adds a new help field under the text and leaves the existing help field there. I suspect a few people such as myself have looked at the module, installed it, and wondered why it doesn't work!
Use Field group module
A Field group (Drupal 7 only) can have a description, which is displayed immediately below the field group label. So wrap your field in a group and, if necessary, theme away any unwanted markup (such as the label of the contained field).
Rather than put a group around a single field you may find, as I did, that grouping a couple of fields together, with a description covering both, has advantages for usability.
Thank you.
Thank you.
Your observation really helped me. I needed to add aditional information about a field group that had lots of content, this helped.
Thank you.
Markup
If there are only a few fields that need the help text, I add a markup field right above the corresponding field.