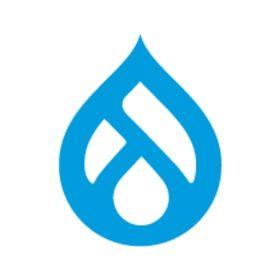
In the implementation of a test for #562932: {filter_format}.cache is not saved, I need to check if the cache was cleared correctly when filters were added/changed/reordered in a text format.
Currently, the test is doing something like this:
$result = db_query('SELECT * FROM {cache_filter}')->fetchObject();
$this->assertFalse($result, t('Filter cache cleared.'));
The problem I see here is that we cannot assume that the cache subsystem being used is the default (database tables, DrupalDatabaseCache class). So, we need an API function to check if a cache bin is empty.
A cache bin might be considered empty if it does not contain any valid (non expired) data for any cache ID.
Attached an initial patch:
- Added cache_bin_is_empty($bin) API function to check if a cache bin is empty .
- Added isEmpty() method to DrupalCacheInterface.
- Implemented isEmpty() in DrupalDatabaseCache class.
Comment | File | Size | Author |
---|---|---|---|
#14 | cache-is-empty-14.patch | 3.78 KB | dropcube |
#11 | cache-is-empty-11.patch | 3.31 KB | dropcube |
#10 | cache-is-empty-10.patch | 3.36 KB | dropcube |
#7 | cache-is-empty-7.patch | 1.51 KB | dropcube |
#4 | cache-is-empty-4.patch | 1.51 KB | dropcube |
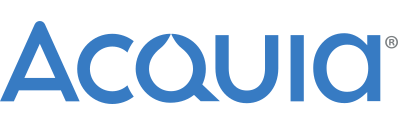
Comments
Comment #1
dropcube CreditAttribution: dropcube commenteduf, removed eclipse project stuff from the patch.
Comment #2
Dries CreditAttribution: Dries commentedWhy do we use the word _bin_ in the function name? I think this should be called cache_is_empty() for consistency.
Comment #3
catchShouldn't this be a db_query_range($query, 0, 1);?
Comment #4
dropcube CreditAttribution: dropcube commentedAs suggested above:
- Changed the function name to cache_is_empty()
- Used db_query_range()
Comment #5
sunTrailing white-space and misaligned PHPDoc comment here.
Shouldn't this be a count query or similar?
If that first row by coincidence happens to be big, then we process a lot of data for nothing.
Please remove the trailing blank line after ::isEmpty().
I'm on crack. Are you, too?
Comment #6
Damien Tournoud CreditAttribution: Damien Tournoud commentedSee http://drupal.org/update/modules/6/7#select_count for how this query should be written.
Comment #7
dropcube CreditAttribution: dropcube commented- Fixed coding style issues.
- Used a count query as described at http://drupal.org/update/modules/6/7#select_count
Comment #8
Damien Tournoud CreditAttribution: Damien Tournoud commentedThe docblock doesn't match the function parameters.
This has to be a dynamic query, because the table changes.
Something like this should work:
This review is powered by Dreditor.
Comment #9
Damien Tournoud CreditAttribution: Damien Tournoud commentedNever write DB:TNG from memory... the correct query is probably more:
Comment #10
dropcube CreditAttribution: dropcube commented- Used dynamic query.
- Fixed parameters in PHPDoc.
- Added tests.
Comment #11
dropcube CreditAttribution: dropcube commentedforgot to remove the comments, here it's
Comment #13
CorniI CreditAttribution: CorniI commentedPlease add an implemenation to includes/cache-install.inc DrupalFakeCache class which was committed a minute ago...
Comment #14
dropcube CreditAttribution: dropcube commented- Added DrupalFakeCache::isEmpty() method.
Comment #15
sunHm - auto-running GC looks like some (perhaps?) unwanted magic to me. Not sure though.
Missing trailing comma after last array element.
Can we squeeze a blank line between the chunks/chapters here? :)
This review is powered by Dreditor.
Comment #16
Dries CreditAttribution: Dries commentedI'm happy with this now, and it cleans up some of the existing APIs which is Slush-worthy. Committed to CVS HEAD. Thanks.
Comment #17
dropcube CreditAttribution: dropcube commented@sun
There might be a case, when the bin only contains expired entries. So, once the garbage collector has run, the bin is actually empty. As the comments say, a cache bin is considered empty if it does not contain any *valid* data for any cache ID.
Comment #18
andypostLooks like this change is breaks alternative cache-backends (memcache, apc...) because it's not possible to count items in most of them.
Is this API change is really required?
Comment #19
catchWe have wildcard cache clearing in our API as well, but neither support that, I think it's OK for them to just silently not work for things like this - better than querying cache tables directly when you are using the default backend.
Comment #20
dropcube CreditAttribution: dropcube commentedIn this case, alternative cache-backends could use flags or any other approach to know if a cache bin is empty. For example, when a bin is flushed, set the flag to empty, then when data is set, change the flag to non-empty, etc...
Comment #21
chx CreditAttribution: chx commentedor, to be one the safe side you can always report as nonempty, worst can happen is a test fail, big deal.