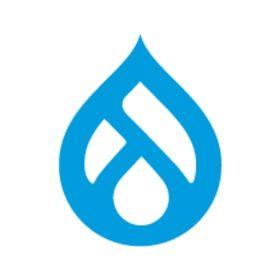
- Count your forum containers ...
- Using a text editor, create a Java script file, gave it a name, for example: collapse-containers.js using the following code, and put it in the /misc folder :
" Note : the following code is for 11 containers , count the containers in your forum and place the corresponding definitions after the first line "var containers = { };" of the Java script code. If you want to make new containers later , you need to edit this file in the future "
var containers = { }; containers["container_1"] = { "show" : 1 }; containers["container_2"] = { "show" : 1 }; containers["container_3"] = { "show" : 1 }; containers["container_4"] = { "show" : 1 }; containers["container_5"] = { "show" : 1 }; containers["container_6"] = { "show" : 1 }; containers["container_7"] = { "show" : 1 }; containers["container_8"] = { "show" : 1 }; containers["container_9"] = { "show" : 1 }; containers["container_10"] = { "show" : 1 }; containers["container_11"] = { "show" : 1 }; function elwardah_forum_class_filter(r, m) { m = " " + m + " "; var tmp = []; for (var i = 0; r[i]; i++) { var pass = (" " + r[i].className + " ").indexOf(m) >= 0; if (pass) { tmp.push(r[i]); } } return tmp; } function elwardah_forum_toggle_display(m) { containers[m]["show"] = !containers[m]["show"]; elwardah_forum_render(m); elwardah_forum_store_preferences(); }; function elwardah_forum_render(m) { var rows = document.getElementsByTagName("tr"); rows = elwardah_forum_class_filter(rows, m); for ( var i = 0; rows[i]; i++ ) { if (containers[m]["show"]) { elwardah_forum_show(rows[i]); } else { elwardah_forum_hide(rows[i]); } } var c = document.getElementById(m); var e = c.getElementsByTagName("span"); e = elwardah_forum_class_filter(e, "show_hide_message"); e[0].innerHTML = containers[m]["show"] ? "Hide" : "Show"; } function elwardah_forum_hide(e) { e.oldblock = e.oldblock || e.style.display; if (e.oldblock == "none") { e.oldblock = "block"; } e.style.display = "none"; } function elwardah_forum_show(e) { e.style.display = e.oldblock ? e.oldblock : ""; if (e.style.display == "none") { e.style.display = "block"; } } function elwardah_forum_store_preferences() { var data = []; for (var c in containers) { data.push(c + ":" + Number(containers[c]["show"])); } data = escape(data.join(";")); var date = new Date(); date.setTime(date.getTime() + (365*24*60*60*1000)); var expires = "; expires=" + date.toGMTString(); document.cookie = "forumprefs=" + data + expires + "; path=/"; } function elwardah_forum_load_preferences() { var cookieName = "forumprefs="; var ca = document.cookie.split(";"); for(var i = 0; i < ca.length; i++) { var c = unescape(ca[i].replace(/^\s+|\s+$/g, "")); if (c.indexOf(cookieName) == 0) { var data = c.substring(cookieName.length, c.length); data = data.split(";"); for (var k = 0; data[k]; k++) { var pair = data[k].split(":"); if (containers[pair[0]]) { containers[pair[0]]["show"] = Number(pair[1]); } } } } for (var c in containers) { elwardah_forum_render(c); } } function elwardah_stop_bubble(e) { if (e.stopPropagation) e.stopPropagation(); e.cancelBubble = true; }
- Using a text editor, create a file and give it the name "forum_list.tpl.php" using the following code. Put it in your active theme folder.
<?php /** * This snippet generates the forum_list layout. * This works with Drupal 4.7 and Drupal 5.x * For use with a custom forum_list.tpl.php file. * */ global $user; // checks the current user so later the snippet can determine what permissions they have if ($forums) { // check if there is forums. drupal_add_js("misc/collapse-containers.js"); // include the Java script file in the pages that has forums $container_flag = 0; foreach ($forums as $key=>$forum) { // this is the start of the forum list routine that generates the containers and forum list. if ($forum->container) { // If the forum is a container if ($container_flag) { // I wanted each container to be in a separate table print theme('table', $header, $rows); $rows = $nrows; } $container_flag += 1; $description = "<div>\n"; $description .= ' <div class="name">'. l($forum->name, "forum/$forum->tid/container") ."</div>\n"; if ($forum->description) { $description .= " <div class=\"description\">$forum->description</div>\n" ; } $description .= "<span class=\"show_hide_message\">Show</span>"."</div>\n"; $rows[] = array( 'class' => 'container', 'data' => array('data' => array( 'data' => $description,'colspan' =>4)), 'id'=>'container_'.$container_flag, 'onclick'=>"elwardah_forum_toggle_display(this.id);" ); // this sets the column titls and style sheet class names $rows[] = array('class' => 'container_'.$container_flag, 'data' =>array( array('data' => t('Subject'), 'class' => 'f-subject'), array('data' => t('Topics'), 'class' => 'f-topics'), array('data' => t('Posts'),'class' => 'f-posts'), array('data' => t('Last post'),'class' => 'f-last-reply') )); } else { // If the forum is not a container $forum->old_topics = _forum_topics_unread($forum->tid, $user->uid); if ($user->uid) { $new_topics = _forum_topics_unread($forum->tid, $user->uid); } else { $new_topics = 0; } $description = '<div>'; $description .= ' <div class="name">'. l($forum->name, "forum/$forum->tid") ."</div>\n"; if ($forum->description) { $description .= " <div class=\"description\">$forum->description</div>\n"; } $description .= "</div>\n"; $rows[] = array( 'class' => 'container_'.$container_flag, 'data' =>array( array('data' => $description, 'class' => 'forum'), array('data' => $forum->num_topics . ($new_topics ? '<br>'. l(format_plural($new_topics, '1 new', '@count new'), "forum/$forum->tid", NULL, NULL, 'new') : ''), 'class' => 'topics'), array('data' => $forum->num_posts, 'class' => 'posts'), array('data' => _forum_format($forum->last_post), 'class' => 'last-reply'))); } } /** * Only set the table header if page is a container with listing of forums */ if(in_array(arg(1), variable_get('forum_containers', array()))){ // reverse array $nrows = array_reverse($rows,true); //get the container description $container = taxonomy_get_term(arg(1)); //add to output $rows = $nrows; $rows[] = array( 'class' => 'container_'.$container_flag, 'data' =>array( array('data' => t('Subject'), 'class' => 'f-subject'), array('data' => t('Topics'), 'class' => 'f-topics'), array('data' => t('Posts'),'class' => 'f-posts'), array('data' => t('Last post'),'class' => 'f-last-reply') )); $rows[] = array( 'class' => 'container', 'data' => array('data' => array( 'data' => $description,'colspan' =>4)), 'id'=>'container_'.$container_flag, 'onclick'=>"elwardah_forum_toggle_display(this.id);" ); //reverse again to output $nrows = array_reverse($rows,true); $rows = $nrows; } print theme('table', $header, $rows); } ?>
- In a text editor, create a new file called "template.php" and copy the following snippet into the file. If a "template.php" file already exists, simply add the snippet remembering to omit the opening and closing PHP tags (i.e.
and
)<?php /** * This snippet tells Drupal to override the forum_list function * and load a custom forum_list.tpl.php layout file * in the theme folder */ function phptemplate_forum_list($forums, $parents, $tid) { return _phptemplate_callback('forum_list', array('forums' => $forums, 'parents' =>$parents, 'tid' =>$tid)); } ?>
- Make the required addition to your css file to stylish the "show_hide_message" class, for example:
show_hide_message { float: left; color:#fff; font-size:1.6em; font-weight:bold; }
- The result can be found here :
Note : The idea was inspired by the forum of :
Comments
Porting this to Drupal 6.x
*Closing EDIT 2008-08-06* I don't have a clue how to monitor this comment, so it will be discontinued. The development of this feature is being developed for the advanced forum module here: http://drupal.org/node/291084
Hi, does anybody know how to port this to Drupal 6.x followed the directions, without luck.
*EDIT* 2008-07-23: Some fixes for 6.x below.
If you use advanced forum, you need to follow this fix: http://drupal.org/node/260633. Okay great! You will get a nasty error from forum_list.tpl.php on the content: array('data' => _forum_format($forum->last_post), 'class' => 'last-reply')));
You need to fix it by replacing it with: array('data' => ($forum->last_reply), 'class' => 'last-reply')));
as stated here: http://drupal.org/node/237412
This will work in some way, It would be cool if advanced forum adapted this. It would greatly improve Drupal Forums with the integration of collapsible forum containers.
/grn
GrN.dk
creating collapsible containers in drupal 6.12
I am new bee to the drupal I want the drupal collapsible container as shown in the website http://software.intel.com/en-us/forums/ so please clearly expalin wher to keep the to keep the code in a particular region
with reards
Ugesh