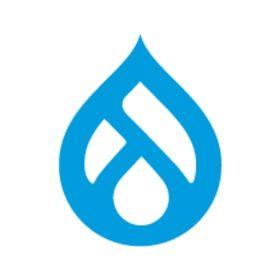
I had to place profile module's fields in my own form.
Thanks to this blog http://echodittolabs.org/profile-module-form-wrapper, I managed to do it for Drupal 6.
Here is a snippet :)
function my_module_menu() {
$items['my_module/form'] = array(
'title' => 'My form',
'page callback' => 'my_module_form',
'access arguments' => array('access content'),
'description' => 'My form',
'type' => MENU_CALLBACK,
);
return $items;
}
function my_module_form() {
return drupal_get_form('my_module_profile_form','Personal Information');
}
/*
WRAPPER FUNCTIONS FOR PROFILE.MODULE FORM
The profile form is usually only available on the user editing form.
Some wrapper functions are necessary to make it work elsewhere.
*/
function my_module_profile_form($user,$category)
{ global $user;
if(module_exists('profile'))
{
$user = user_load(array('uid' => $user->uid));
$edit = array();
foreach($user as $key => $value)
if(preg_match('/^profile_/',$key))
$edit[$key] = $value;
$form = profile_form_profile($edit, $user, $category);
// now let's pretend we're in hook_form_alter
// add a submit button
$form['op'] = array
(
'#type' => 'submit',
'#value' => t('Submittt'),
'#weight' => 30
);
// pass along the $category parameter as a hidden value
$form['category'] = array
(
'#type' => 'hidden',
'#value' => $category,
);
return $form;
}
}
function my_module_profile_form_submit($form, &$form_state)
{
global $user;
if(module_exists('profile'))
{
$r = profile_save_profile($form_state['values'], $user, $form_state['values']['category']);
// reload the user with the newly-saved info
$user = user_load(array('uid' => $user->uid));
drupal_set_message(t('Your order was saved.'));
return $r;
}
}
Comments
List selection options snippet
Here is a snippet to pull the options from a profile field select list into an array for use in your forms. Use this if you're building a form in a module, and want to populate your forms options with data from the profile module (specifically a list type, with options):
You can then put the $options array into your form definition like so:
I hope this helps someone in the position I was in a couple hours ago ;)